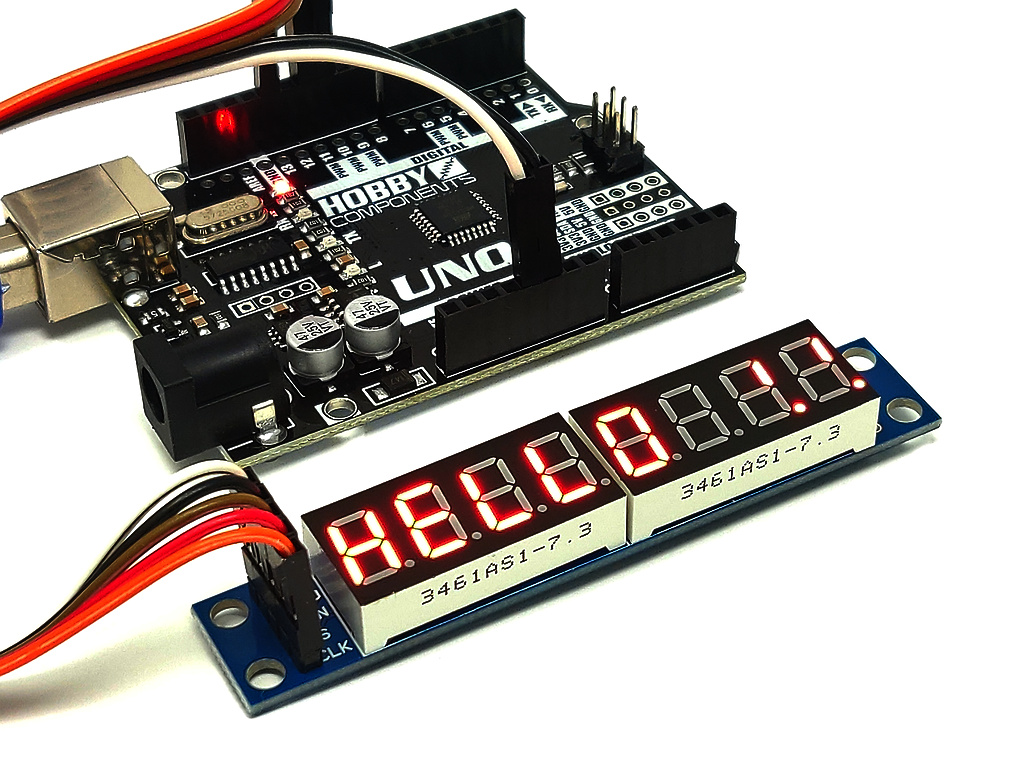

This 8 digit seven segment display module (HCMODU0260) is based upon the Maxim MAX7219 LED display driver. The module uses two standard 4 digit seven segment displays to display a total of 8 digits in RED with decimal point. A convenient input and output header allows additional modules to be daisy-chained and controlled with just just 3 digital IO pins. For panel mounting, the module has 4 mounting holes.
The Maxim MAX7219 driver allows for each segment of up to 8 digits (with decimal point) to be controlled individually from a serial interface. An 8x8 static ram area stores each digit and multiplexing the display is handled automatically.
If you plan to use this module with an Arduino board we have written an exclusive library (HCMAX7219) than allows you to effortlessly display and scroll alphanumeric characters across one or more modules. This can be downloaded from the software section of our support forum here:
viewforum.php?f=58
The module is supplied with unsoldered 1x 4 way right angle and 1x way straight pin headers.
HOBBY COMPONENTS NOTES:
PLEASE NOTE WHEN CONNECTING MULTIPLE MODULES: The modules are fitted with a reverse polarity protection diode in series with the 5V (VCC) supply. This has the effect of reducing the voltage on the VCC output pin relative to the voltage applied to its VCC input pin. Therefore when daisy-chaining multiple modules you should either supply 5V to each module's VCC input pin separately, or remove the diode (D1) on each additional module.
Specification
Model number: HCMODU0160 Driver: Maxim MAX7921 Voltage: 4.0 - 5.5V Max current: 180mA (all LEDs on) Min current: <0.5mA (shutdown mode) Length: 82.3mm Width: 15mm Height: 11.5mm (approx) Display length: 61mm Display width: 14.3mm

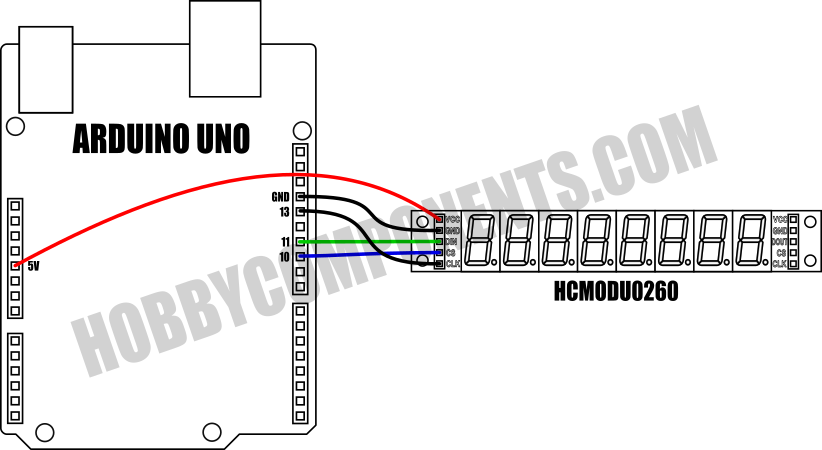
- /* FILE: HCMODU0082_Serial_7_Segment_Module_Example2
- DATE: 19/03/15
- VERSION: 0.2
- REVISIONS:
- 12/03/15 Created version 0.1
- 19/03/15 Updated to work with V0.2 of the HCMAX7219 library
- This is an example of how to use the Hobby Components serial 8 digit seven 7
- segment display module (HCMODU0082). To use this example sketch you will
- need to download and install the HCMAX7921 library available from the software
- section of our support forum (forum.hobbycomponents.com) or on github:
- (https://github.com/HobbyComponents)
- The library assumes you are using one module. If you have more than one module
- connected together then you will need to change the following line in the
- libraries HCMAX7219.h header file to the number of drivers you have connected:
- #define NUMBEROFDRIVERS 1 <- Change this number
- PINOUT:
- MODULE.....UNO/NANO.....MEGA
- VCC........+5V..........+5V
- GND........GND..........GND
- DIN........11...........51
- CS (LOAD)..10...........10
- CLK........13...........52
- You may copy, alter and reuse this code in any way you like, but please leave
- reference to HobbyComponents.com in your comments if you redistribute this code.
- This software may not be used directly for the purpose of promoting products that
- directly compete with Hobby Components Ltd's own range of products.
- THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO WARRANTIES,
- WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED
- WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR
- LACK OF NEGLIGENCE. HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE
- FOR ANY DAMAGES INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR
- CONSEQUENTIAL DAMAGES FOR ANY REASON WHATSOEVER. */
- /* Include the HCMAX7219 and SPI library */
- #include <HCMAX7219.h>
- #include "SPI.h"
- /* Set the LOAD (CS) digital pin number*/
- #define LOAD 10
- /* Create an instance of the library */
- HCMAX7219 HCMAX7219(LOAD);
- void setup()
- {
- HCMAX7219.Init();
- }
- /* Main program */
- void loop()
- {
- byte Loopcounter;
- byte Position;
- /* SCROLL SOME TEXT 5 TIMES BEFORE MOVING ON */
- for (Loopcounter = 0; Loopcounter <= 5; Loopcounter++)
- {
- /* We are scrolling 30 characters of text across the entire display */
- for (Position = 0; Position <= DISPLAYBUFFERSIZE + 30; Position++)
- {
- /* Write the test to the output buffer at the position we require */
- HCMAX7219.print7Seg("HCMAX7219 SCROLLING TEXT DEMO ",Position);
- /* Send the output buffer to the display */
- HCMAX7219.Refresh();
- delay(200);
- }
- }
- /* WE CAN ALSO DISPLAY INTEGER NUMBERS */
- /* Clear the output buffer */
- HCMAX7219.Clear();
- /* Write some text and output it*/
- HCMAX7219.print7Seg("INT NUM.",8);
- HCMAX7219.Refresh();
- delay(2000);
- /* display an example of a negative integer number */
- HCMAX7219.Clear();
- HCMAX7219.print7Seg(-1234567,8);
- HCMAX7219.Refresh();
- delay(2000);
- /* Clear the output buffer */
- HCMAX7219.Clear();
- /* Write some text and output it*/
- HCMAX7219.print7Seg("WITH DP.",8);
- HCMAX7219.Refresh();
- delay(2000);
- /* Show the DP in different places. Notice when the DP is at the
- beginning the number is padded with a zero */
- HCMAX7219.Clear();
- for (Position = 1; Position <= 7; Position++)
- {
- HCMAX7219.print7Seg(-1234567,Position,8);
- HCMAX7219.Refresh();
- delay(1000);
- }
- }

HCMAX7921 Arduino library for above sketch is available for download from the software section of our support forum here:
http://forum.hobbycomponents.com/viewto ... =58&t=1794
Or from github here:
https://github.com/HobbyComponents/HCMAX7219
MAX7921 Datasheet:
Libraries, example code, and diagrams are provided as an additional free service by Hobby Components and are not sold as part of this product. We do not provide any guarantees or warranties as to their accuracy or fitness for purpose.