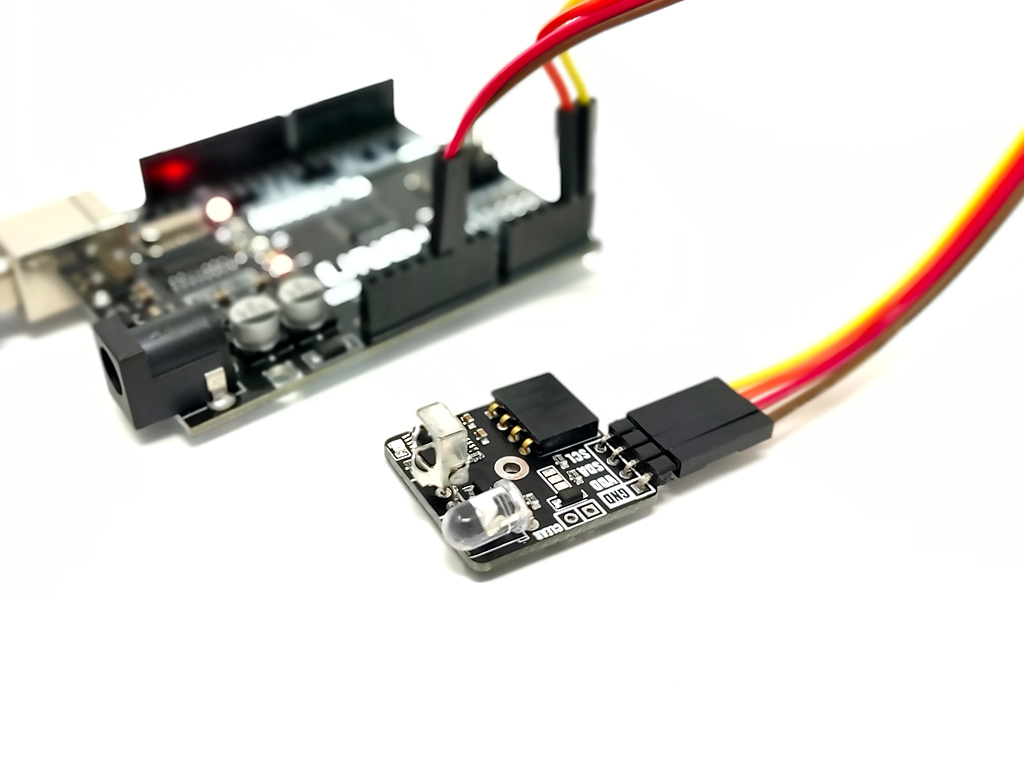

The mLink NEC IR Transceiver is a small serial I2C based module that is capable of sending and receiving infrared remote control signals compatible with the NEC protocol.
The NEC IR protocol is the prevalent standard for infrared remote communication, widely adopted by various IR-enabled devices like TVs, set-top boxes, air conditioners, and projectors. With this module, you can use your microcontroller to effortlessly capture and transmit signals to and from these compatible devices, making it an excellent choice for home automation projects.
Compatible with microcontrollers equipped with an I2C interface, including popular Arduino models, wireless ESP devices, and Raspberry Pi, this module offers versatile control options. As part of the mLink series, it seamlessly integrates with other mLink modules. This means you can easily daisy-chain multiple mLink modules, enabling centralised control through a single serial interface.
For Arduino users you can use the mLink library (see below for library and examples) to control any type of mLink module. Only one single instance of the library is needed to control multiple types of mLink modules resulting in very little resource overhead and therefore making it great for Arduinos with small amounts of memory and pin counts.
For Raspberry Pi users we have a Python module which can be installed via pip or downloaded and installed directly from our forum. Please see the mLink Python forum thread for requirements and download link here: viewtopic.php?f=131&t=3062&p=8592#p8592
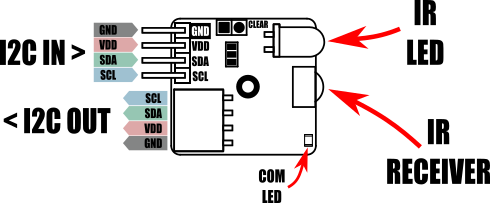

Module code: HCMODU0195 Supply voltage (VDD): 5V Current consumption (idle): 6mA I2C Interface speed: 400kbits/s (fast mode) I2C default address (HEX): 0h5C Maximum number of modules: 5 with pullups fitted, 112 with pullups removed* IR modulation frequency: 38KHz IR reception angle: ~45 degrees IR reception distance (max): 18m IR transmission range (max): 8m IR compatible protocol: NEC infrared protocol Module dimensions ex headers (LxWxH): 24.5mm x 23mm x 13mm
*Note the maximum number of connected modules will depend on cable lengths and power requirements of each module. Do not exceed 5 mLink modules connected in series with I2C pullups fitted to all modules. For disconnecting pullups see forum post.
Arduino Connection Example:
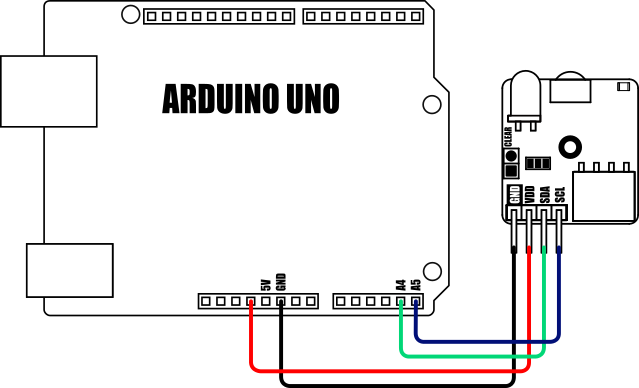
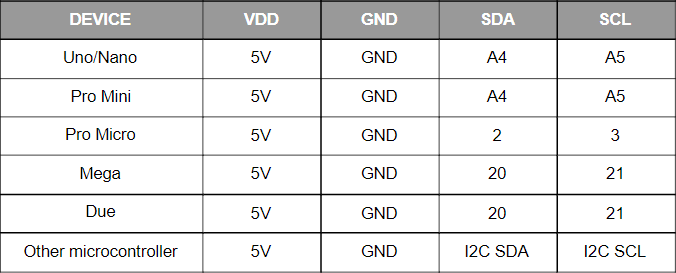
Raspberry Pi Connection Example:
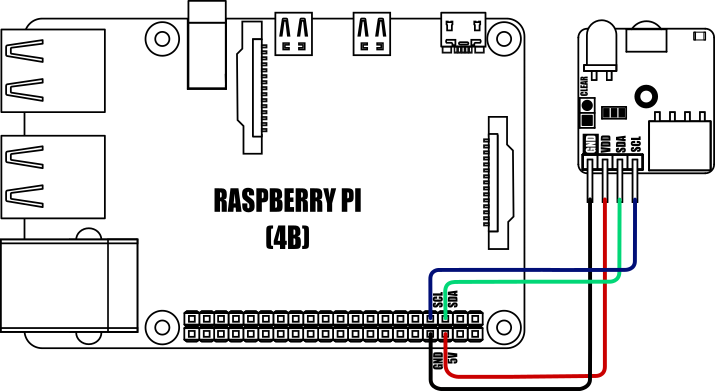
Other useful information:
Factory Reset:
Should you wish to restore the module back to its factory default configuration, this can be done by manually forcing a factory reset. All mLink modules include a set of pads labelled clear:
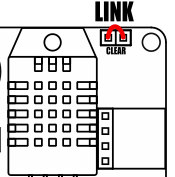
To perform a factory reset carefully link the two pads together with a piece of wire or with something conductive such as a paperclip. Whilst linked, connect power to the module via the VCC and GND connections. Wait a few seconds and then remove the short from the pads. The module's settings, including its I2C address, should now be restored back to factory defaults.
Removing I2C pullups:
If your development board already has pullups for its I2C interface fitted, or you wish to connect more than 5 mLink boards to the same I2C interface, then you can remove the pullups for the additional boards by cutting the tracks between the solder pads shown below.
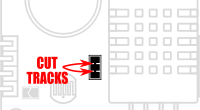

mLink Arduino library
viewtopic.php?f=58&t=3001
Please note you will need at least V1.7.1 (20 Oct 2023) of the library to support this module. If you have an older version already installed please update it to the latest version.
mLink Raspberry Pi Python module
The mLink python module can be installed with the following terminal command:
- pip install hc-mlink
Alternatively the library can be manually installed by downloading it from the forum and unzipping it to your project folder. See the Python module forum thread for more information and download link:
viewtopic.php?f=131&t=3062&p=8592#p8592
Please note that in some cases there may be additional configuration required. If you have issues getting your Raspberry Pi to communicate with the mLink module then please see the Python module forum thread here: viewtopic.php?f=131&t=3062
mLink Specifications and Register Map
https://hobbycomponents.com/downloads/m ... er_Map.pdf
Libraries, example code, and diagrams are provided as an additional free service by Hobby Components and are not sold as part of this product. We do not provide any guarantees or warranties as to their accuracy or fitness for purpose.