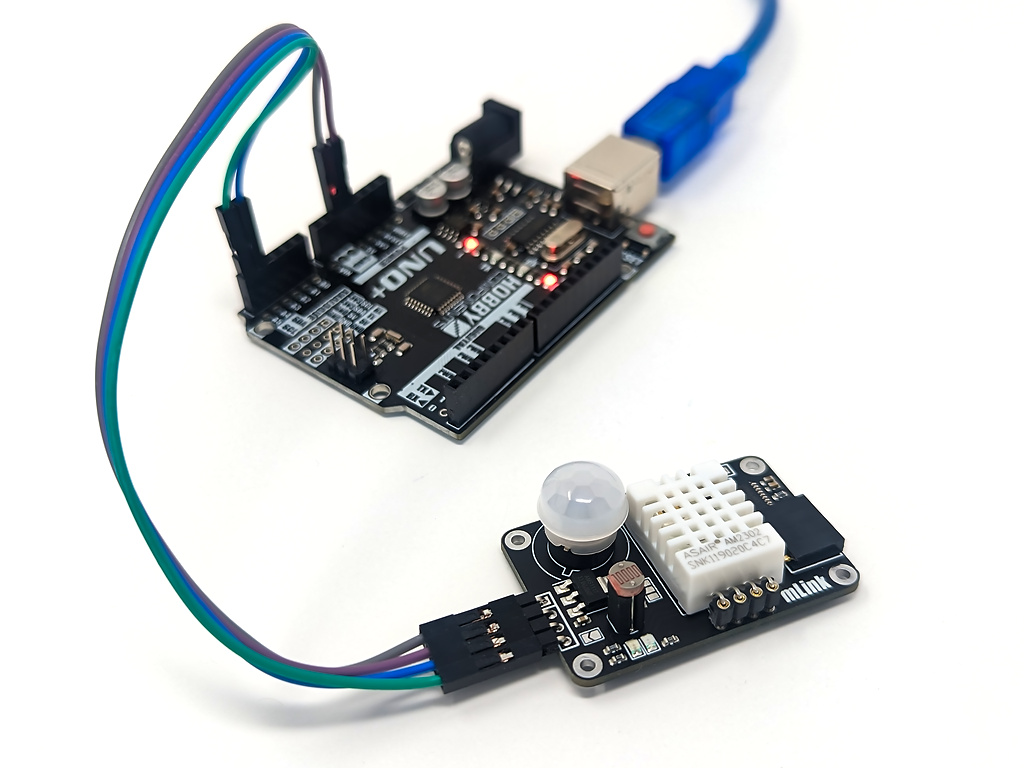

The mLink Home sensor is a compact multiple sensor module featuring a DHT22 temperature/humidity sensor, a LDR light sensor and a PIR motion detector. These features make this module ideal for sensing occupancy and environmental conditions within a room.
Communication with the sensors is made via the modules serial (I2C) interface which is compatible with microcontrollers featuring an I2C interface including most types of Arduinos. Being an mLink module it is also compatible with other modules in our mLink range and can simply be daisy-chained together allowing multiple different mLink modules to be controlled via one serial interface.
For Arduino users you can use the mLink library (see below for library and examples) to control any type of mLink module. Only one single instance of the library is needed to control multiple types of mLink modules resulting in very little resource overhead and therefore making it great for Arduinos with small amounts of memory and pin counts.
For Raspberry Pi users we have a Python module which can be installed via pip or downloaded and installed directly from our forum. Please see the mLink Python forum thread for requirements and download link here: viewtopic.php?f=131&t=3062&p=8592#p8592
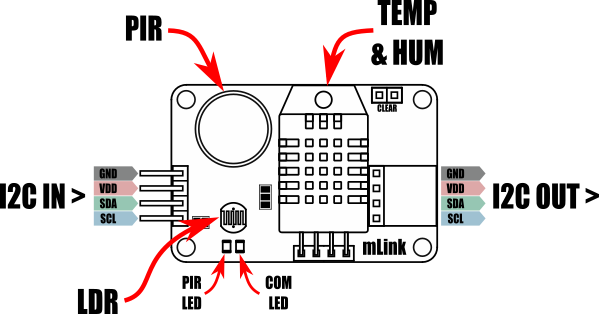

Module code: HCMODU0198 Supply Voltage(VDD): 5V (3.3V with solder pad bridged - see forum post) Current consumption: 7mA Current consumption (sleep): 0.5mA I2C Interface speed: 400kbits/s (fast mode) I2C default address (HEX): 0h5B Maximum number of modules: 5 with pullups fitted, 112 with pullups removed* Temperature sensor range: -40 to 80oC Temperature sensor resolution: 0.1oC Humidity sensor range: 0 to 10 %RH Humidity sensor resolution: 0.1 %RH LDR sensor range: 0 to 255 PIR Trigger on time: ~4 seconds PIR Sensor angle: 100 degree cone Sensor range: ~3 to 5 metres Module dimensions ex headers (LxWxH): 45mm x 30mm x 18mm
*Note the maximum number of connected modules will depend on cable lengths and power requirements of each module. Do not exceed 5 mLink modules connected in series with I2C pullups fitted to all modules. For disconnecting pullups see forum post.
Arduino Connection Example:
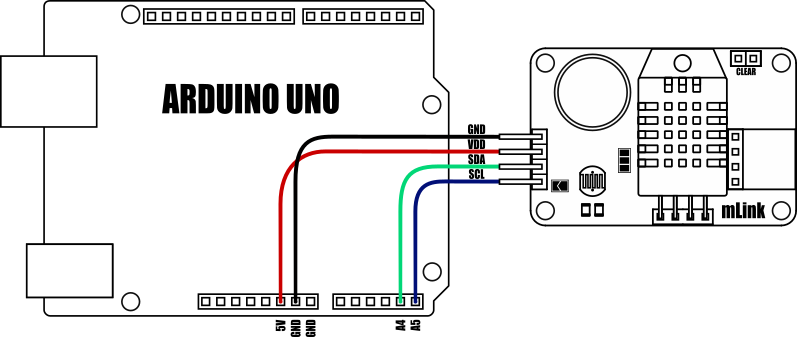
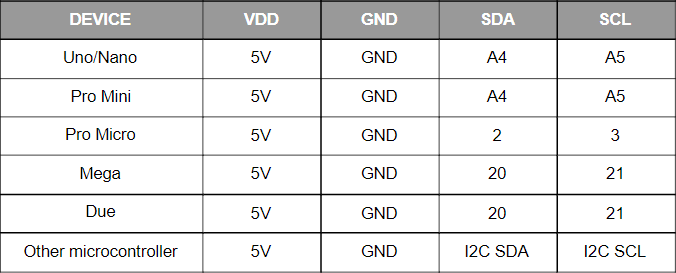
Because the modules use an I2C interface this also means multiple modules can be controlled from a single Arduino I2C interface simply by daisy-chaining them together. Note to control multiple mLink modules of the same type requires changing the default I2C address of the additional modules. See example Arduino sketch on forum post.

Raspberry Pi Connection Example:
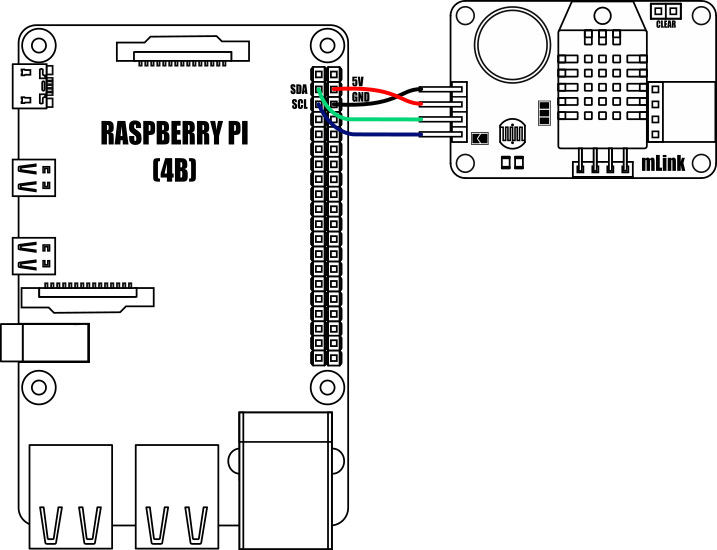
Other useful information:
Factory Reset:
Should you wish to restore the module back to its factory default configuration, this can be done by manually forcing a factory reset. All mLink modules include a set of pads labelled clear:
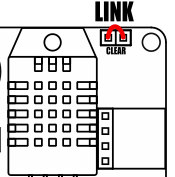
To perform a factory reset carefully link the two pads together with a piece of wire or with something conductive such as a paperclip. Whilst linked, connect power to the module via the VCC and GND connections. Wait a few seconds and then remove the short from the pads. The module's settings, including its I2C address, should now be restored back to factory defaults.
Removing I2C pullups:
If your development board already has pullups for its I2C interface fitted, or you wish to connect more than 5 mLink boards to the same I2C interface, then you can remove the pullups for the additional boards by cutting the tracks between the solder pads shown below.
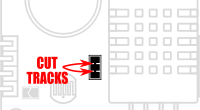
3.3V operation
If you wish to power this board via a 3.3V power supply you can bypass the onboard 5V regulator by linking the following pad with a solder bridge.
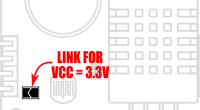
Once linked, the VDD input header pin will now require a 3.3V supply instead of 5V.
Note: When configured for a 3.3V supply this module can only be connected to additional mLink modules that are 3.3V compatible.
Dimensions:
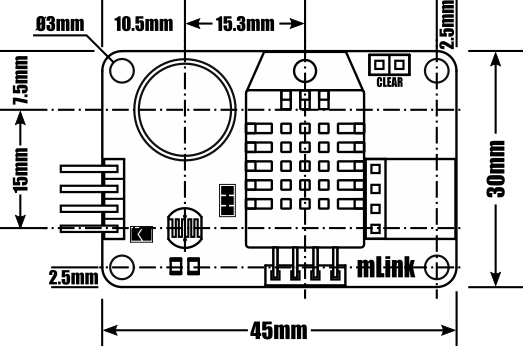

mLink Arduino library
viewtopic.php?f=58&t=3001
Please note you will need at least V1.6 (07 Jan 2023) of the library to support this module. If you have an older version already installed please update it to the latest version.
mLink Raspberry Pi Python module
The mLink python module can be installed with the following terminal command:
- pip install hc-mlink
Alternatively the library can be manually installed by downloading it from the forum and unzipping it to your project folder. See the Python module forum thread for more information and download link:
viewtopic.php?f=131&t=3062&p=8592#p8592
Please note that in some cases there may be additional configuration required. If you have issues getting your Raspberry Pi to communicate with the mLink module then please see the Python module forum thread here: viewtopic.php?f=131&t=3062
mLink Specifications and Register Map
https://hobbycomponents.com/downloads/m ... er_Map.pdf
Libraries, example code, and diagrams are provided as an additional free service by Hobby Components and are not sold as part of this product. We do not provide any guarantees or warranties as to their accuracy or fitness for purpose.