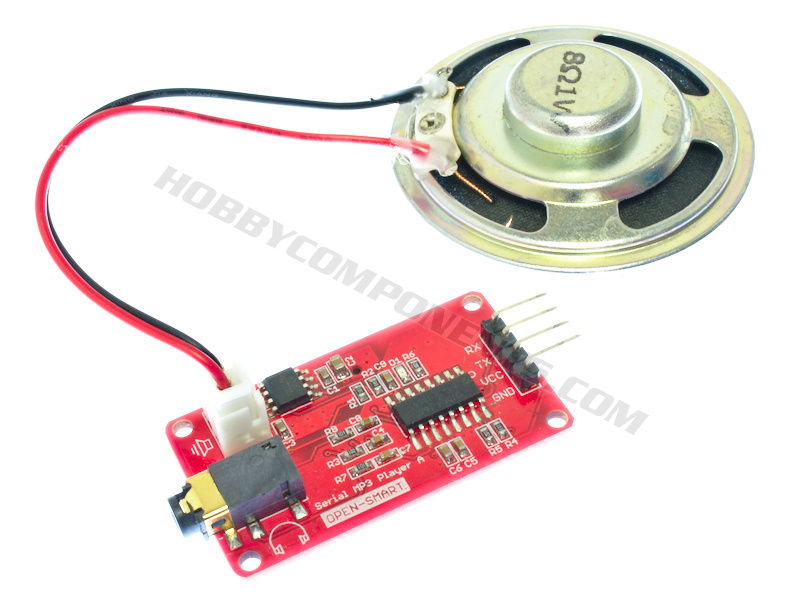

This modules is a simple MP3 player device which is based on a high-quality MP3 audio chip. It can support 8k Hz ~ 48k Hz sampling frequency MP3 and WAV file formats. There is a TF card socket on board, so you can plug in the micro SD card that stores audio files. MP3 Playback can be controlled via a Microcontroller such as an Arduino by sending commands to the module via serial UART port, e.g. switch songs, change the volume, play mode and so on. It is compatible with most Microcontrollers capable of serial communication at 9600 BAUD (Arduino / AVR / ARM / PIC). The module also includes a 3 Watt amplifier and comes complete with a 1 Watt speaker.
For Arduino users we have created an exclusive library (HCMP3) which can be downloaded from the software section of our support forum (see link below).
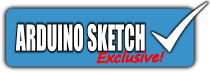
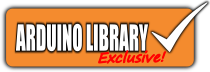
Specification:
- Product code: HCMODU0138
- Compatible with Arduino UNO / Leonardo / Mega2560 / DUE
- Supports sampling frequency (kHz): 8 / 11.025 / 12 / 16 / 22.05 / 24 / 32 / 44.1 / 48
- Supports file formats: MP3 / WAV
- Support Micro SD card, Micro SDHC Card
- On-board 3-watt mono amplifier
- On-board speaker interface XH2.54-2P, can connect to external speakers such as 8 ohm 1 to 3-watt
- 30 class adjustable volume
- UART TTL serial control playback mode, baud rate is 9600bps
- Serial communication format: 8N1
- Control logic interface can be 3.3V / 5V TTL
- Working voltage: 3.7 - 5.25VDC
- On-board headphone jack for connection to headphones or external amplifier
- On-board TF card connector
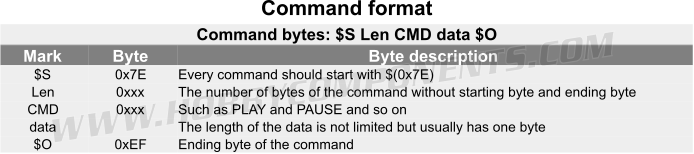
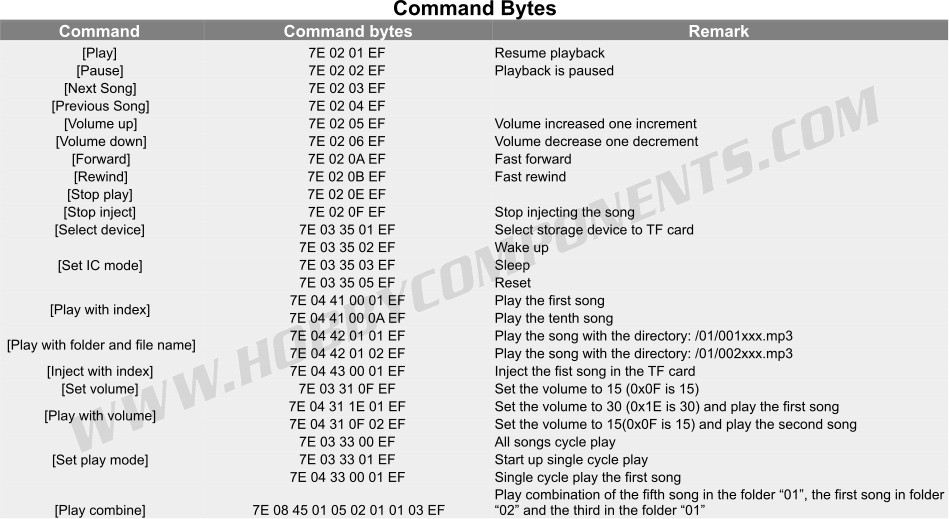

Return bytes from a command status query are preceded with the original status command. For Example:
To get the current play status send the command: 7E 02 10 EF
If the module is currently playing a file it will return: 7E 02 00 EF 7E 03 10 01 EF
If the module is currently paused it will return: 7E 02 00 EF 7E 03 10 02 EF
Example Arduino Sketch Using HCMP3 Library:
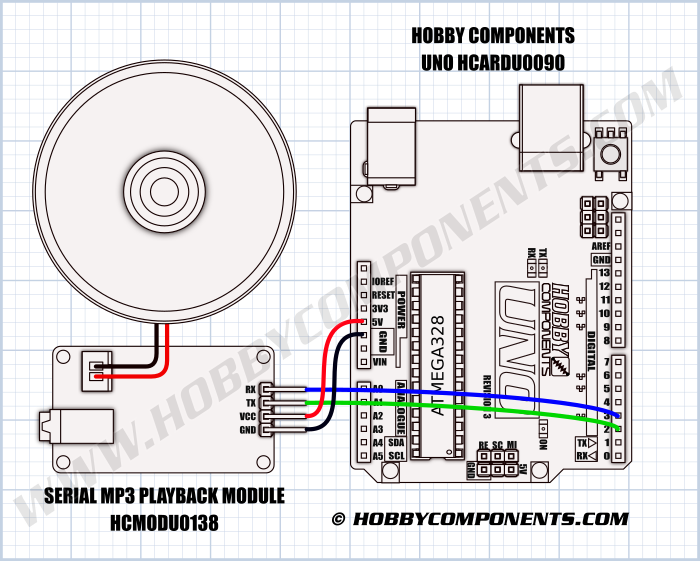
- /* FILE: HCMP3_Example.cpp
- DATE: 09/05/19
- VERSION: 1.0
- AUTHOR: Andrew Davies
- 09/05/19 version 1.0: Original version
- This example sketch demonstrates how to use the HCMP3 library to control
- an audio playback device.
- Currently this library supports the following module:
- Serial MP3 playback module with 1W speaker (SKU: HCARDU0138)
- Connect the above module as follows:
- Arduino.........MP3 Module
- RX..............3
- TX..............2
- VCC.............5V
- GND.............GND
- Within the HCMP3 library folder you will find a folder called 'MP3_Files'
- Copy the contents of this folder to the root directory of your flash card.
- This library is provided free to support the open source community.
- We spend most of our time creating free content like this because we genuinely
- want to support the open source community. PLEASE SUPPORT US so that we can
- continue to create free content by purchasing products from our store -
- HOBBYCOMPONENTS.COM
- You may copy, alter and reuse this code in any way you like, but please leave
- reference to HobbyComponents.com in your comments if you redistribute this code.
- This software may not be used directly for the purpose of selling products that
- directly compete with Hobby Components Ltd's own range of products.
- THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO WARRANTIES, WHETHER
- EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF
- MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR LACK OF NEGLIGENCE.
- HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR ANY DAMAGES,
- INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR ANY
- REASON WHATSOEVER.
- */
- /* Include the library */
- #include "HCMP3.h"
- /* Digital pins used for serial communication */
- #define RX_PIN 2
- #define TX_PIN 3
- /* Create an instance of the library */
- HCMP3 HCMP3(TX_PIN, RX_PIN);
- void setup()
- {
- Serial.begin(9600);
- HCMP3.reset();
- /* Get the current status of the module */
- Serial.println("MODULE STATUS");
- Serial.print("Card state....");
- PrintCardState();
- Serial.print("Playback state.....");
- PrintPlaybackState();
- Serial.print("Volume level....");
- Serial.println(HCMP3.getVolumeState());
- Serial.println("");
- delay(2000);
- /* Change the volume level */
- Serial.print("Setting volume level to....");
- HCMP3.volume(10);
- Serial.println(HCMP3.getVolumeState());
- delay(2000);
- }
- void loop()
- {
- /* Play the first file */
- Serial.println("Playing first file on card");
- HCMP3.play();
- delay(2000);
- /* Play the next file */
- Serial.println("Playing next file");
- HCMP3.playNext();
- delay(2000);
- /* Play the previous file */
- Serial.println("Playing previous file");
- HCMP3.playPrevious();
- delay(2000);
- /* Play the first file in folder 1 */
- Serial.println("Playing file 1 in folder 1");
- HCMP3.play(1,1);
- delay(5000);
- /* Pause playback */
- Serial.print("Pausing....");
- HCMP3.pause();
- PrintPlaybackState();
- delay(2000);
- /* Resume playback */
- Serial.print("Resuming....");
- HCMP3.play();
- PrintPlaybackState();
- delay(2000);
- /* Turn the volume down in increments */
- Serial.print("Volume down");
- for(byte i = 0; i < 20; i++)
- {
- Serial.print(".");
- HCMP3.volumeDown();
- delay(100);
- }
- Serial.println("");
- /* Turn the volume up in increments */
- Serial.print("Volume up");
- for(byte i = 0; i < 20; i++)
- {
- Serial.print(".");
- HCMP3.volumeUp();
- delay(100);
- }
- Serial.println("");
- delay(5000);
- /* Stop the playback */
- Serial.print("Playback...");
- HCMP3.stop();
- PrintPlaybackState();
- while(1);
- }
- /* Prints out the current playback state */
- void PrintPlaybackState()
- {
- switch(HCMP3.getPlayState())
- {
- case(STATUS_STOP):
- Serial.println("Stopped");
- break;
- case(STATUS_PLAY):
- Serial.println("Playing");
- break;
- case(STATUS_PAUSED):
- Serial.println("Paused");
- break;
- }
- }
- /* Prints out the current flash card state */
- void PrintCardState()
- {
- switch(HCMP3.getCardState())
- {
- case(CARD_EJECTED):
- Serial.println("Ejected");
- break;
- case(CARD_INSERTED):
- Serial.println("Inserted");
- break;
- case(CARD_UNKOWN):
- Serial.println("Unknown");
- break;
- }
- }

The HCMP3 Arduino library for the above sketch can be downloaded from the software section of our support forum here:
http://forum.hobbycomponents.com/viewto ... =58&t=2884
Disclaimer: Libraries, example code, and diagrams within this forum thread are provided as an additional free service by Hobby Components and are not sold as part of any product. We do not provide any guarantees or warranties as to their accuracy or fitness for purpose.
Descriptions and diagrams on this page are copyright Hobby Components Ltd and may not be reproduced without permission.