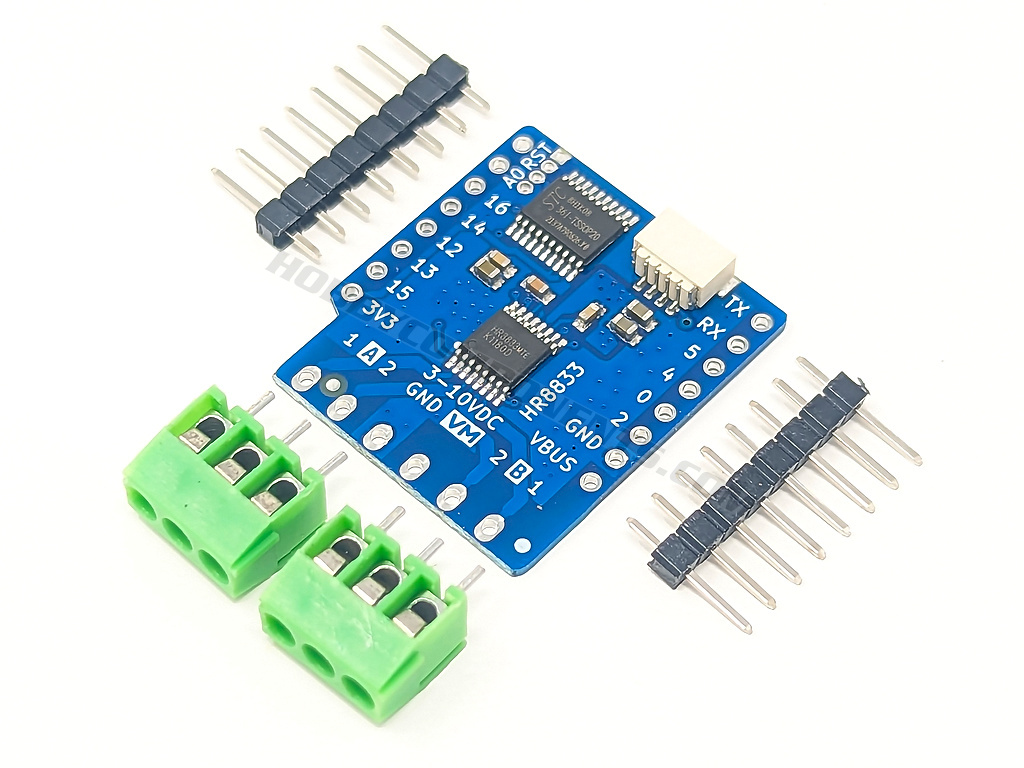

The WeMos I2C Dual Motor Driver Module is designed to be able to drive up to two DC motors from your WeMos/Lolin mini or mini Pro development boards (See HCWEMO0002). Using PWM the shield is capable of independently controlling the speed and direction of each connected motor with a maximum average of up to 1.2A (3.2A peak). The shield, together with its supplied headers (2 x 8 way supplied unsoldered), allows for it to be easily inserted and removed from the WeMos Mini. Control of the shield is via the WeMos mini's I2C interface (0x30 default address). A library and example sketch is provided by WeMos to make controlling the shield from your own sketches as simple as possible.
Features
I2C interface
Power supply voltage: VM=15V max
Output current: Iout=1.2A(average) / 3.2A (peak)
Standby control to save power
CW/CCW/short brake/stop motor control modes
Pins
D1 mini GPIO Shield D1 5 SCL D2 4 SDA RST RST RST VM: Motor power supply + (Max 15Vdc) GND: Motor power supply - 1-A-2: Motor A 2-B-1: Motor B
LOLIN V2.0 Shield Arduino sketch (See underside of board for version number)
- /*
- Simple Motor Shield Test.
- */
- #include <Wire.h>
- #include <LOLIN_I2C_MOTOR.h>
- LOLIN_I2C_MOTOR motor; //I2C address 0x30
- // LOLIN_I2C_MOTOR motor(DEFAULT_I2C_MOTOR_ADDRESS); //I2C address 0x30
- // LOLIN_I2C_MOTOR motor(your_address); //using customize I2C address
- void setup()
- {
- Serial.begin(115200);
- Serial.println("Motor Shield Testing...");
- while (motor.PRODUCT_ID != PRODUCT_ID_I2C_MOTOR) //wait motor shield ready.
- {
- motor.getInfo();
- }
- }
- void loop()
- {
- Serial.println("Change A to CCW, B to CW, Freq: 1000Hz");
- Serial.println("Duty Tesing...");
- motor.changeFreq(MOTOR_CH_BOTH, 1000); //Change A & B 's Frequency to 1000Hz.
- /*
- motor.changeFreq(MOTOR_CH_A, 1000);//Change A 's Frequency to 1000Hz.
- motor.changeFreq(MOTOR_CH_B, 2000);//Change B 's Frequency to 2000Hz.
- */
- motor.changeStatus(MOTOR_CH_A, MOTOR_STATUS_CCW);
- motor.changeStatus(MOTOR_CH_B, MOTOR_STATUS_CW);
- for (float duty = 0; duty <= 100; duty++)
- {
- motor.changeDuty(MOTOR_CH_A, duty);
- motor.changeDuty(MOTOR_CH_B, 100 - duty);
- Serial.print("Change A Duty to ");
- Serial.print(duty);
- Serial.println("%");
- Serial.print("Change B Duty to ");
- Serial.print(100 - duty);
- Serial.println("%");
- delay(100);
- }
- Serial.println("STANDBY Tesing...");
- motor.changeStatus(MOTOR_CH_BOTH, MOTOR_STATUS_CCW);
- motor.changeDuty(MOTOR_CH_BOTH, 100);
- for (int i = 0; i < 5; i++)
- {
- Serial.println("MOTOR_STATUS_STANDBY");
- motor.changeStatus(MOTOR_CH_BOTH, MOTOR_STATUS_STANDBY);
- delay(500);
- Serial.println("MOTOR_STATUS_CW");
- motor.changeStatus(MOTOR_CH_BOTH, MOTOR_STATUS_CW);
- delay(500);
- Serial.println("MOTOR_STATUS_STANDBY");
- motor.changeStatus(MOTOR_CH_BOTH, MOTOR_STATUS_STANDBY);
- delay(500);
- Serial.println("MOTOR_STATUS_CCW");
- motor.changeStatus(MOTOR_CH_BOTH, MOTOR_STATUS_CCW);
- delay(500);
- }
- Serial.println("MOTOR_STATUS Tesing...");
- for (int i = 0; i < 5; i++)
- {
- Serial.println("MOTOR_STATUS_STOP");
- motor.changeStatus(MOTOR_CH_A, MOTOR_STATUS_STOP);
- delay(500);
- Serial.println("MOTOR_STATUS_CCW");
- motor.changeStatus(MOTOR_CH_A, MOTOR_STATUS_CCW);
- delay(500);
- Serial.println("MOTOR_SHORT_BRAKE");
- motor.changeStatus(MOTOR_CH_A, MOTOR_STATUS_SHORT_BRAKE);
- delay(500);
- Serial.println("MOTOR_STATUS_CW");
- motor.changeStatus(MOTOR_CH_A, MOTOR_STATUS_CW);
- delay(500);
- }
- }

Downloads for Lolin V.20 shield (see bottom of shield to confirm the version you have)
Schematic:
Arduino Library:
The library can also be downloaded from the following GitHub page:
https://github.com/wemos/LOLIN_I2C_MOTOR_Library
Libraries, example code, and diagrams are provided as an additional free service by Hobby Components and are not sold as part of this product. We do no provide any guarantees or warranties as to their accuracy or fitness for purpose.
Descriptions and diagrams on this page are copyright Hobby Components Ltd and may not be reproduced without permission.