
The ULN2003 Stepper Motor Driver Board uses a ULN2003 IC to drive 4-phase, 5-wire stepper motors.
Supply Voltage: 5V (Supplied motor suitable for 5V applications)
Max Output Current: 0.5A (Single Output)
4 LED indicators
Size: 40.5mm x 21.3mm
Stepper Motor Cable Length (approx.): 24cm
Holding Torque: 0.3kg/cm
Diameter: 28mm
Step Angle: 5.625 degrees
Reduction Ratio: 1/64
Pinout
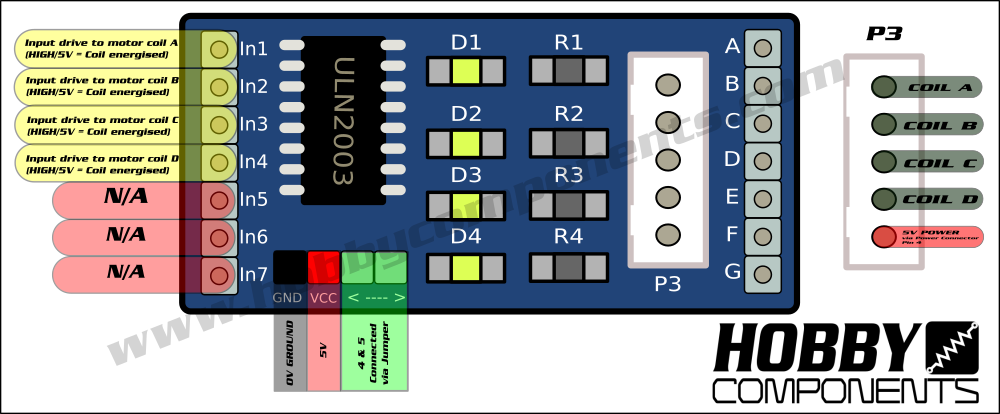
Example Arduino Sketch
- /* FILE: ARD_Stepper_Motor_HCROBO0055_Example.pde
- DATE: 02/09/12
- VERSION: 0.1
- This is an example of how to use the HobbyComponents Arduino compatible stepper
- motor with driver interface (HCROBO0055) . When run this program will
- continually
- rotate the motor one full revolution in the forward direction
- followed by one full
- revolution in the reverse direction. Although this stepper motor will
- work with the
- standard Arduino stepper motor library functions, this program
- demonstrates how easy
- it is to directly control.
- You may copy, alter and reuse this code in any way you like, but please leave
- reference to HobbyComponents.com in your comments if you redistribute
- this code.
- THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO
- WARRANTIES, WHETHER
- EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED
- WARRANTIES OF
- MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR LACK
- OF NEGLIGENCE.
- HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR ANY DAMAGES,
- INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR CONSEQUENTIAL
- DAMAGES FOR ANY
- REASON WHATSOEVER.
- */
- /* Defines the values passed to the function vStepMotor()
- to specify which direction you would like the motor to
- turn in. */
- #define FORWARD true
- #define REVERSE false
- /* Defines the digital IO pins used to control each of
- the stepper motors coils. */
- #define MOTOR_COIL_1_DIO 8
- #define MOTOR_COIL_2_DIO 9
- #define MOTOR_COIL_3_DIO 10
- #define MOTOR_COIL_4_DIO 11
- /* The number of steps for 1 revolution of this stepper
- motor is 360 degrees divided by the motor step angle
- of 5.625 multiplied by the gear ratio 1/64 multiplied
- by the number of coils 4 divided by two.
- Therefore: 360 / (5.625 * (1 / 64)) = 512 */
- #define STEPSPER1REV 512
- /* Set the 4 DIO pins used to drive the stepper motor to outputs */
- void setup()
- {
- pinMode(MOTOR_COIL_1_DIO, OUTPUT);
- pinMode(MOTOR_COIL_2_DIO, OUTPUT);
- pinMode(MOTOR_COIL_3_DIO, OUTPUT);
- pinMode(MOTOR_COIL_4_DIO, OUTPUT);
- }
- /* MAIN PROGRAM */
- void loop()
- {
- /* Used for counting the number of steps the motor has made */
- word u16StepCounter;
- /* Rotate the stepper motor one full forward revolution */
- for (u16StepCounter = 0; u16StepCounter < STEPSPER1REV; u16StepCounter++)
- {
- vStepMotor(FORWARD, 3);
- }
- /* Rotate the stepper motor one full reverse revolution */
- for (u16StepCounter = 0; u16StepCounter < STEPSPER1REV; u16StepCounter++)
- {
- vStepMotor(REVERSE, 3);
- }
- }
- /* Function will pulse all four coils of the stepper motor in either the forward
- or reverse directions. The function accepts two inputs, a true or a false denoting
- the direction the motor should turn in (true = forward, false =reverse), and a step
- delay time in milliseconds between each step. */
- void vStepMotor(boolean bDirection, word u16StepDelay)
- {
- /* Holds which DIO pin is currently associated with which motor coil */
- byte bMotorCoil_1;
- byte bMotorCoil_2;
- byte bMotorCoil_3;
- byte bMotorCoil_4;
- /* Set the order of the DIO pins depending on the direction the
- motor is requires to turn in. */
- if (bDirection == true)
- {
- bMotorCoil_1 = MOTOR_COIL_1_DIO;
- bMotorCoil_2 = MOTOR_COIL_2_DIO;
- bMotorCoil_3 = MOTOR_COIL_3_DIO;
- bMotorCoil_4 = MOTOR_COIL_4_DIO;
- }else
- {
- bMotorCoil_1 = MOTOR_COIL_4_DIO;
- bMotorCoil_2 = MOTOR_COIL_3_DIO;
- bMotorCoil_3 = MOTOR_COIL_2_DIO;
- bMotorCoil_4 = MOTOR_COIL_1_DIO;
- }
- /* Pulse each of the stepper motors coils in a sequential order */
- digitalWrite(bMotorCoil_1, HIGH);
- digitalWrite(bMotorCoil_2, LOW);
- digitalWrite(bMotorCoil_3, LOW);
- digitalWrite(bMotorCoil_4, LOW);
- delay(u16StepDelay);
- digitalWrite(bMotorCoil_1, LOW);
- digitalWrite(bMotorCoil_2, HIGH);
- digitalWrite(bMotorCoil_3, LOW);
- digitalWrite(bMotorCoil_4, LOW);
- delay(u16StepDelay);
- digitalWrite(bMotorCoil_1, LOW);
- digitalWrite(bMotorCoil_2, LOW);
- digitalWrite(bMotorCoil_3, HIGH);
- digitalWrite(bMotorCoil_4, LOW);
- delay(u16StepDelay);
- digitalWrite(bMotorCoil_1, LOW);
- digitalWrite(bMotorCoil_2, LOW);
- digitalWrite(bMotorCoil_3, LOW);
- digitalWrite(bMotorCoil_4, HIGH);
- delay(u16StepDelay);
- digitalWrite(bMotorCoil_1, LOW);
- digitalWrite(bMotorCoil_2, LOW);
- digitalWrite(bMotorCoil_3, LOW);
- digitalWrite(bMotorCoil_4, LOW);
- }
Downloads:
Disclaimer: Libraries, example code, and diagrams are provided as an additional free service by Hobby Components and are not sold as part of this product. We do no provide any guarantees or warranties as to their accuracy or fitness for purpose.
Descriptions and diagrams on this page are copyright Hobby Components Ltd and may not be reproduced without permission.