
The DS05-NFC high torque servo (HCROBO0050) is able to rotate continuously in full 360 rotations in both forward (clockwise) and reverse (anti-clockwise) directions. It will accept standard servo signals for its input meaning it can be driven from just one 5V digital pin of a microcontroller. A pulse-width of 1ms will turn the servo at full reverse speed, and 2ms pulse width will turn the servo at full forward speed. Any pulse width between these two will proportionally set the speed between full forwards and backwards rotation.
The servo is also supplied with accessories including fixing screws and several servo arms (see image).

Item number: HCROBO0050
Model number: DS05-NFC
Weight: 39g
Body dimensions: 40.8 x 20 x 39.5mm
Torque: 5.5kg/cm (at 4.8V)
Speed: 0.22sec/60° (at 4.8V)
Operating voltage: 4.8v - 5.5v
Operating temperature: 0°C - 60oC
Current: < 1000mA
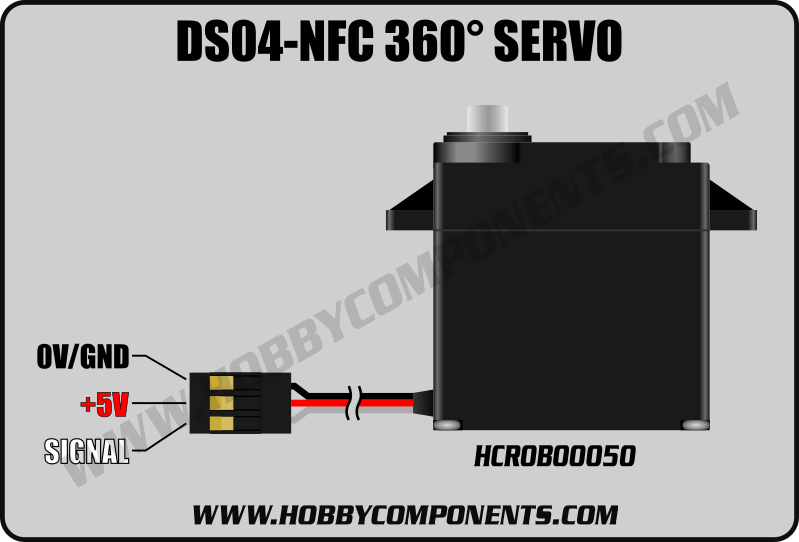
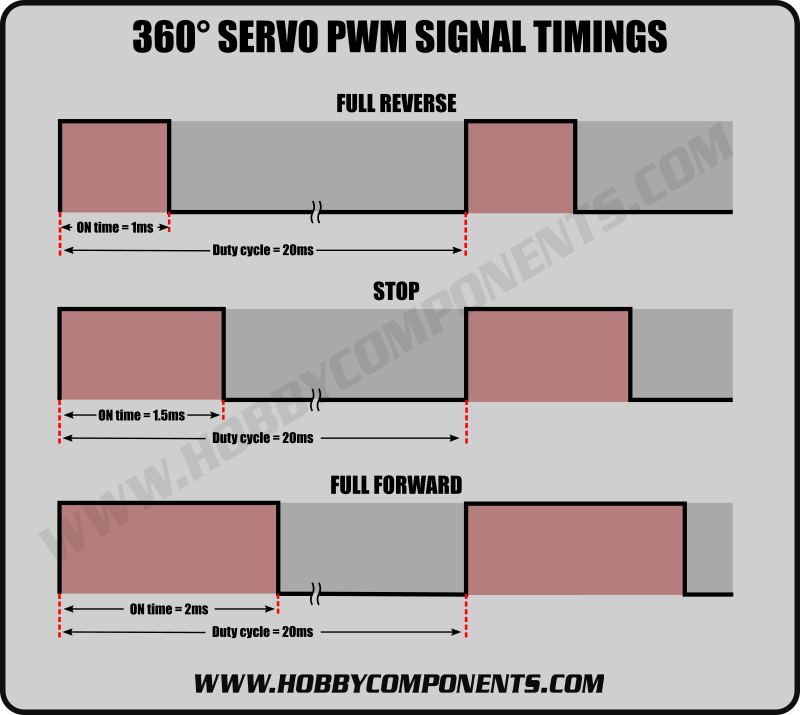

Example Using The Arduino Servo Library:
Code: Select all
/* FILE: 360_Degree_Servo_Example
DATE: 13/01/16
VERSION: 0.1
AUTHOR: Andrew Davies
13/01/16 version 0.1: Original version
Example to drive a 360 degree servo in both directions using the standard Arduino
Servo library. To use this example you must connect the signal pin of the servo
to your Arduino's digital pin 8
You may copy, alter and reuse this code in any way you like, but please leave
reference to HobbyComponents.com in your comments if you redistribute this code.
This software may not be used directly for the purpose of selling products that
directly compete with Hobby Components Ltd's own range of products.
THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO WARRANTIES, WHETHER
EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF
MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR LACK OF NEGLIGENCE.
HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR ANY DAMAGES,
INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR ANY
REASON WHATSOEVER.
*/
/* Include the servo library */
#include <Servo.h>
/* Servo will be attached to digital pin 8 */
#define SERVOPIN 8
/* Create an instance of the servo library */
Servo servo;
void setup()
{
/* Attach the servo to the digital pin */
servo.attach(SERVOPIN);
}
void loop()
{
/* Servo stopped */
servo.writeMicroseconds(1500);
delay(1000);
/* Servo forward half speed */
servo.writeMicroseconds(1750);
delay(1000);
/* Servo forward full speed */
servo.writeMicroseconds(2000);
delay(1000);
/* Servo forward half speed */
servo.writeMicroseconds(1750);
delay(1000);
/* Servo stopped */
servo.writeMicroseconds(1500);
delay(1000);
/* Servo backwards half speed */
servo.writeMicroseconds(1250);
delay(1000);
/* Servo backwards full speed */
servo.writeMicroseconds(1000);
delay(1000);
/* Servo backwards half speed */
servo.writeMicroseconds(1250);
delay(1000);
}
Simple Bit Bashing Example:
Code: Select all
/* FILE: 360_Degree_Servo_Example_No_Library
DATE: 13/01/16
VERSION: 0.1
AUTHOR: Andrew Davies
13/01/16 version 0.1: Original version
Example to drive a 360 degree servo in both directions. It demonstrates the use of
very simple bit bashing instead of using the the Arduino servo library. To use this
example you must connect the signal pin of the servo to your Arduino's digital pin 8.
You may copy, alter and reuse this code in any way you like, but please leave
reference to HobbyComponents.com in your comments if you redistribute this code.
This software may not be used directly for the purpose of selling products that
directly compete with Hobby Components Ltd's own range of products.
THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO WARRANTIES, WHETHER
EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF
MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR LACK OF NEGLIGENCE.
HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR ANY DAMAGES,
INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR ANY
REASON WHATSOEVER.
*/
/* Servo will be attached to digital pin 8 */
#define SERVOPIN 8
void setup()
{
/* Set the digital pin to an output */
pinMode(SERVOPIN, OUTPUT);
}
void loop()
{
/* Servo stopped */
servo(SERVOPIN, 0, 1000);
/* Servo forward half speed */
servo(SERVOPIN, 250, 1000);
/* Servo forward full speed */
servo(SERVOPIN, 500, 1000);
/* Servo forward half speed */
servo(SERVOPIN, 250, 1000);
/* Servo stopped */
servo(SERVOPIN, 0, 1000);
/* Servo backwards half speed */
servo(SERVOPIN, -250, 1000);
/* Servo backwards full speed */
servo(SERVOPIN, -500, 1000);
/* Servo backwards half speed */
servo(SERVOPIN, -250, 1000);
}
/* This function will rotate the servo for a set amount of time */
void servo(byte pin, int speed, unsigned int time)
{
if(speed > 500)
speed = 500;
if(speed < -500)
speed = -500;
time = time / 20;
for(time; time > 0; time--)
{
servoPulse(pin, speed);
}
}
/* Send a 1 duty cycle (20ms) pulse to the servo with the required on time*/
void servoPulse(byte pin, int speed)
{
digitalWrite(pin,HIGH);
delayMicroseconds(1500 + speed);
digitalWrite(pin,LOW);
delayMicroseconds(500 - speed);
delay(18);
}