High Accuracy DS3231 RTC & EEPROM (HCMODU0094)
Posted: Tue Jan 12, 2016 4:40 pm

The I2C real time clock module (HCMODU0094) is based on the Maxim high accuracy DS3231 real time clock controller. Using an internal temperature compensation crystal oscillator this RTC IC is able to provide extremely high accurate time keeping. The module includes an on-board battery backup and maintains accurate timekeeping when main power to the device is interrupted. The RTC maintains seconds, minutes, hours, day, date, month, and year information. The date at the end of the month is automatically adjusted for months with fewer than 31 days, including corrections for leap year. The clock operates in either the 24-hour or 12-hour format with an AM/PM indicator. Two programmable time-of-day alarms and a programmable square-wave output are provided. Address and data are transferred serially
through an I2C bidirectional bus. A precision temperature-compensated voltage reference and comparator circuit monitors the status of VCC to detect power failures, to provide a reset output, and to automatically switch to the backup supply when necessary.
Included on this module is an Atmel 24C32 32K (4096 x 8) serial I2C EEPROM which allows the module to be used as a simple data logger storing up to 4K of 8 bit data.
If you plan to use this module with a standard Arduino then you can take advantage of our exclusive library (HCRTC) to quickly integrate this module into your own Arduino project. The library also provides the ability to read and write to the included EEPROM.
Features (RTC):
Highly Accurate RTC Completely Manages All Timekeeping Functions
Real-Time Clock Counts Seconds, Minutes, Hours, Date of the Month, Month, Day of the Week, and Year, with Leap-Year Compensation Valid Up to 2100
Accuracy ±2ppm from 0°C to +40°C
Digital Temp Sensor Output: ±3°C Accuracy
Register for Aging Trim
Two Time-of-Day Alarms
Programmable Square-Wave Output Signal
Simple Serial Interface Connects to Most Microcontrollers
Fast (400kHz) I2C Interface
Battery-Backup Input for Continuous Timekeeping
2.3V to 5.5V Operation
Operating Temperature Ranges: 0°C to +70°C
Features (EEPROM):
Low-Voltage and Standard-Voltage Operation 2.7 (VCC = 2.7V to 5.5V)
Internally Organized 8192 x 8
2-Wire Serial Interface
Schmitt Trigger, Filtered Inputs for Noise Suppression
Bidirectional Data Transfer Protocol
100 kHz (1.8V, 2.5V, 2.7V) and 400 kHz (5V) Clock Rate
32-Byte Page Write Mode (Partial Page Writes Allowed)
Self-Timed Write Cycle (10 ms max)
High Reliability Endurance: 1 Million Write Cycles Data Retention: 100 Years
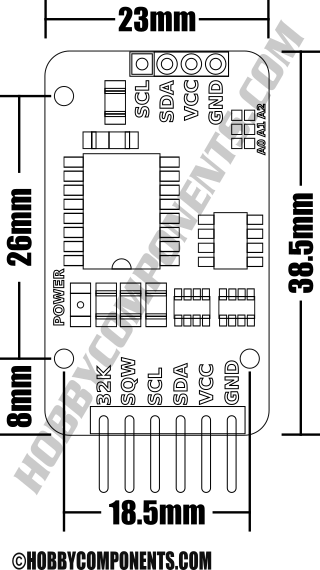

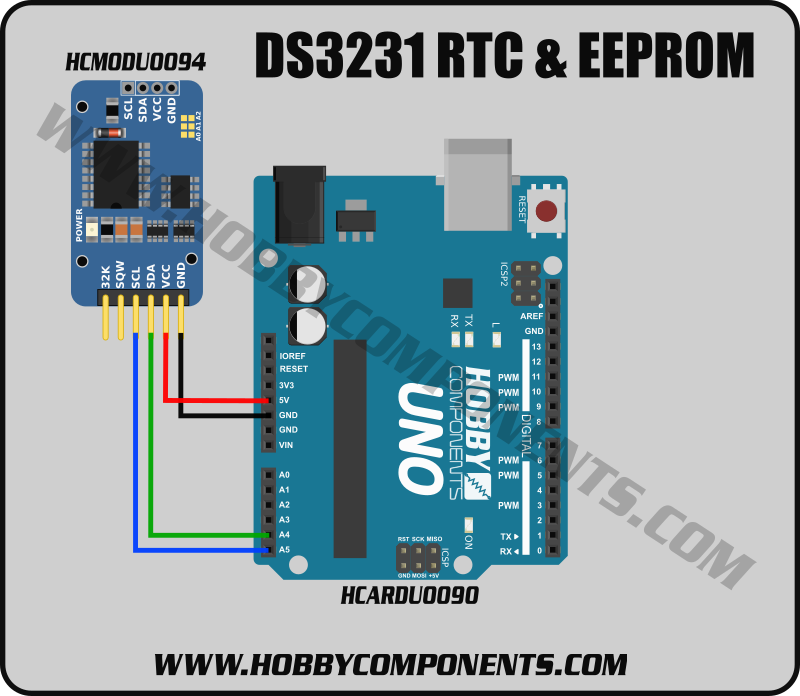
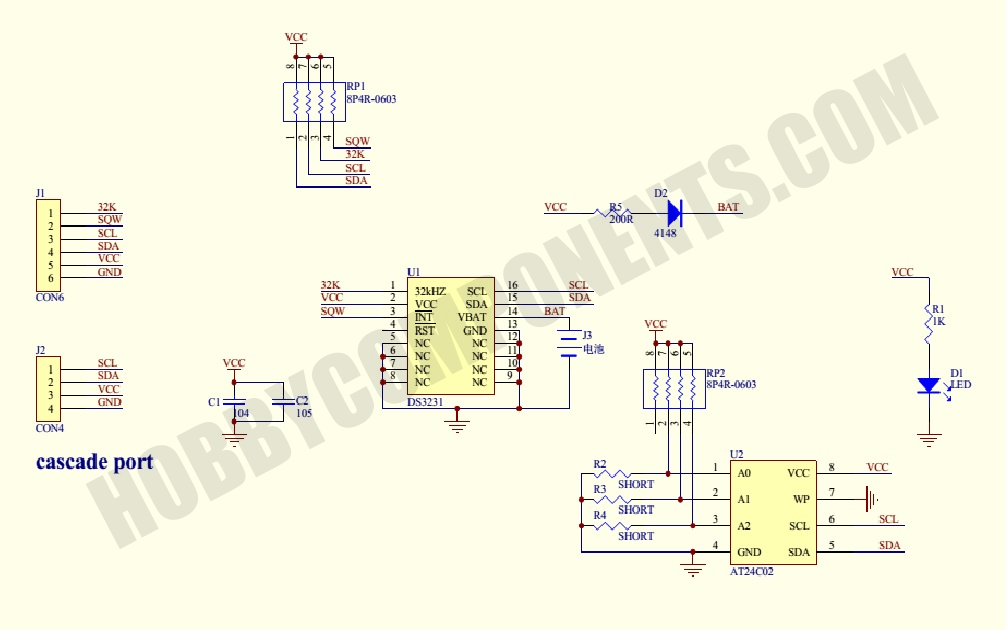

- /* FILE HCRTC_Example.cpp
- DATE: 23/12/13
- VERSION: 0.2
- AUTHOR: Andrew Davies
- This is an example of how to use the Hobby Components RTC library to read and
- write to and from the DS3231 real time clock & 24C32 EEPROM (HCMODU0094).
- You may copy, alter and reuse this code in any way you like, but please leave
- reference to HobbyComponents.com in your comments if you redistribute this code.
- This software may not be used directly for the purpose of selling products that
- directly compete with Hobby Components Ltd's own range of products.
- THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO WARRANTIES, WHETHER
- EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF
- MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR LACK OF NEGLIGENCE.
- HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR ANY DAMAGES,
- INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR ANY
- REASON WHATSOEVER.
- */
- /* Include the wire library */
- #include "Wire.h"
- /* Include the Hobby Components RTC library */
- #include <HCRTC.h>
- /* Define the I2C addresses for the RTC and EEPROM */
- #define I2CDS3231Add 0x68
- #define I2C24C32Add 0x57
- /* Example string to write to EEPROM */
- char ExampleString[] = "Hobby Components";
- /* Used in example as an index pointer */
- byte index;
- /* Create an instance of HCRTC library */
- HCRTC HCRTC;
- void setup()
- {
- Serial.begin(9600);
- /* Use the RTCWrite library function to set the time and date.
- Parameters are: I2C address, year, month, date, hour, minute, second,
- day of week */
- HCRTC.RTCWrite(I2CDS3231Add, 13, 4, 24, 14, 21, 0, 3);
- }
- void loop()
- {
- /************* Example write to EEPROM ***************/
- /* Initiate a write sequence.
- Parameters are: I2C address, EEPROM start location */
- HCRTC.EEStartWrite(I2C24C32Add, 32000);
- for (index = 0; index < sizeof(ExampleString); index++)
- {
- /* Sequentially write example data to the EEPROM */
- HCRTC.EEWriteByte(ExampleString[index]);
- }
- /* End the write sequence */
- HCRTC.EEEndWrite();
- /* Wait for cached data to finish writing */
- delay(10);
- /************* Example read from EEPROM ***************/
- /* Initiate read sequence.
- Parameters are: I2C address, EEPROM start location */
- HCRTC.EEStartRead(I2C24C32Add, 32000);
- for (index = 0; index < sizeof(ExampleString); index++)
- {
- /* Sequentially read data from EEPROM and output it to the UART */
- Serial.write(HCRTC.EEReadByte(I2C24C32Add));
- }
- Serial.println();
- /* Continuously read the current time and date from the RTC */
- while(true)
- {
- /* Read the current time from the RTC module */
- HCRTC.RTCRead(I2CDS3231Add);
- /* Output the information to the UART */
- Serial.print(HCRTC.GetDay());
- Serial.print("/");
- Serial.print(HCRTC.GetMonth());
- Serial.print("/");
- Serial.print(HCRTC.GetYear());
- Serial.print(" ");
- Serial.print(HCRTC.GetHour());
- Serial.print(":");
- Serial.print(HCRTC.GetMinute());
- Serial.print(":");
- Serial.print(HCRTC.GetSecond());
- Serial.print(" DOW:");
- Serial.println(HCRTC.GetWeekday());
- /* Now output the same thing but using string functions instead: */
- Serial.print(HCRTC.GetDatestring());
- Serial.print(" ");
- Serial.println(HCRTC.GetTimestring());
- /* Wait a second before reading again */
- delay(1000);
- }
- }

Arduino Library:
The HCRTC library can be found within the software section of this forum here
Datasheets: