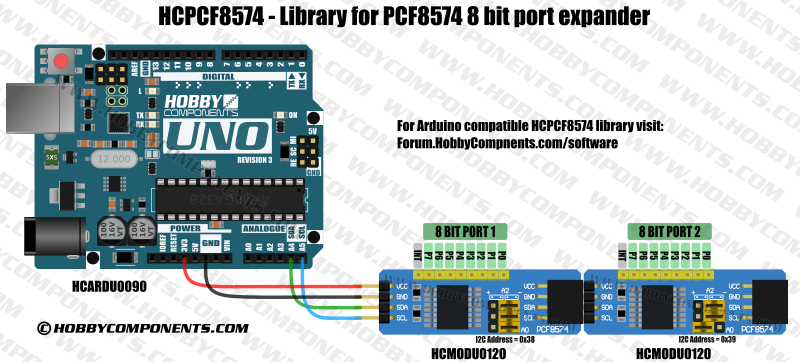

Arduino Library for the PCF8574 I2C to 8-bit digital port expander.
Currently supported products:
PCF8574 I2C to 8-bit digital port expander module (HCMODU0120) available from hobbycomponents.com
This library can be used to configure and control the NXP Semiconductors PCF8571 8-bit digital port expander IC from a standard Arduino development board. Additionally, the library supports the control of multiple devices by creating multiple instances of the library (see HCPCF8574_Multiple_Device_Blink_Example sketch included with library).
You will need to download (please log in to download the library) and unzip this library to the Arduino development environments library area.
On Windows:
My Documents\Arduino\libraries\
On Mac:
Documents/Arduino/libraries/
Linux:
Usually found within the users home area under /Arduino/libraries/
Using the HCPCF8574 library
To use the library just include the HCPCF8574.h header file and then create an instance of the library. E.g:
Code: Select all
#include "HCPCF8574.h"
HCPCF8574 HCPCF8574(I2C_ADD);
To initialise the library add the following line to the setup() section of your sketch:
Code: Select all
HCPCF8574.init();
The following functions are available with this library:
Code: Select all
HCPCF8574.init();
Code: Select all
HCPCF8574.pinMode(Pin, Mode);
Pin is the pin number to configure. Valid values for Pin are 0 to 7
Mode is the required pin direction. Valid values for Mode are
OUTPUT (pin is configured to be an output)
INPUT (pin is configured to be an input)
Note: when configured to an input the pin will be driven high and
masked so that is cannot be written to by the pinWrite function.
Code: Select all
HCPCF8574.pinWrite(Pin, State);
Pin is the number of the pin to alter. Valid values for Pin are 0 to 7
State is the state to set the pin to. Valid values for State are
HIGH (pin is pulled high)
LOW (pin is pulled low)
Note: to read the state of a pin it must be configured as an output by pinMode();
Code: Select all
HCPCF8574.pinRead(Pin);
Pin is the number of the pin to read. Valid values for Pin are 0 to 7
Returns a Boolean value representing the state of the pin.
Note: to read the state of a pin it must be configured as an input by pinMode();
HCPCF8574.portWrite(Data);
Writes to all 8 pins in one operation where:
Data is an 8 bit binary value representing the state of all 8 pins.
Code: Select all
HCPCF8574::portRead();
Returns and 8 bit value representing the state of a 8 pins.

Code: Select all
/* FILE: HCPCF8574_Blink_Example.cpp
DATE: 24/05/17
VERSION: 0.1
AUTHOR: Andrew Davies
24/05/17 version 0.1: Original version
This example sketch uses the HCPCF8574 library to toggle one of the PCF8574's digital pins
emulating the standard Arduino 'blink' sketch. This sketch has been written specifically for
the Hobby Components PCF8574 I2C to 8-bit digital port expander (HCMODU0120). To use the
sketch change the I2C_ADD to match the address of your device (default is 0x38 for HCMODU0120)
and connect and LED (via a current limiting resistor) to the PCF8574's digital pin 0 (marked
D0 on HCMODU0120). You can connect your Arduino to the module as follows:
UNO/NANO........HCMODU0120
VCC.............5V
GND.............GND
A4..............SDA
A5..............SCL
More information about the library can be found in the software section of our support
forum here:
http://forum.hobbycomponents.com/software
You may copy, alter and reuse this code in any way you like, but please leave
reference to HobbyComponents.com in your comments if you redistribute this code.
This software may not be used directly for the purpose of selling products that
directly compete with Hobby Components Ltd's own range of products.
THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO WARRANTIES, WHETHER
EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF
MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR LACK OF NEGLIGENCE.
HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR ANY DAMAGES,
INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR ANY
REASON WHATSOEVER.
*/
#include "HCPCF8574.h" //Include the HCPCF8574 library
#define I2C_ADD 0x38 //I2C address of the PCF8574
HCPCF8574 Port(I2C_ADD); //Create an instance of the library
void setup()
{
Port.init(); //Initiliase the PCF8574 (all pins are set to high)
Port.pinMode(0, OUTPUT); //Set digital pin 0 to an ouput
}
void loop()
{
Port.pinWrite(0, HIGH); //Set digital pin 0 high
delay(1000); //Wait 1 second
Port.pinWrite(0, LOW); //Set digital pin 0 low
delay(1000); //Wait another second
}
Code: Select all
/* FILE: HCPCF8574_Pin_Read_Example.cpp
DATE: 24/05/17
VERSION: 0.1
AUTHOR: Andrew Davies
24/05/17 version 0.1: Original version
This example sketch uses the HCPCF8574 library to read the state of the PCF8574's
digital pin 0 once every second. The state of the pin will then be output to the serial
UART.
This sketch has been written specifically for the Hobby Components PCF8574
I2C to 8-bit digital port expander (HCMODU0120). To use the sketch change the I2C_ADD to
match the address of your device (default is 0x38 for HCMODU0120) and either pull digital
pin 0 high or low to change its state. You can connect your Arduino to the module as follows:
UNO/NANO........HCMODU0120
VCC.............5V
GND.............GND
A4..............SDA
A5..............SCL
More information about the library can be found in the software section of our support
forum here:
http://forum.hobbycomponents.com/software
You may copy, alter and reuse this code in any way you like, but please leave
reference to HobbyComponents.com in your comments if you redistribute this code.
This software may not be used directly for the purpose of selling products that
directly compete with Hobby Components Ltd's own range of products.
THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO WARRANTIES, WHETHER
EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF
MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR LACK OF NEGLIGENCE.
HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR ANY DAMAGES,
INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR ANY
REASON WHATSOEVER.
*/
#include "HCPCF8574.h" //Include the HCPCF8574 library
#define I2C_ADD 0x38 //I2C address of the PCF8574
HCPCF8574 Port(I2C_ADD); //Create an instance of the library
void setup()
{
Serial.begin(9600); //Initiliase the Arduino serial library.
Port.init(); //Initiliase the PCF8574 (all pins are set to high)
Port.pinMode(0, INPUT); //Set digital pin 0 to an input
}
void loop()
{
boolean State = Port.pinRead(0); //Read the state of digital pin 0
// Output the state to the serial port
Serial.print("Pin 0 = ");
if(State)
Serial.println("HIGH");
else
Serial.println("LOW");
delay(1000);
}

Diagrams, libraries, and example code are provided as an additional free service by Hobby Components and are not sold as part of this product. We do not provide any guarantees or warranties as to their accuracy or fitness for purpose.
Descriptions and diagrams on this page are copyright Hobby Components Ltd and may not be reproduced without permission.