Microduino-SD micro SD Card module (HCMIDU0009)
Posted: Sat Jun 01, 2013 7:07 pm
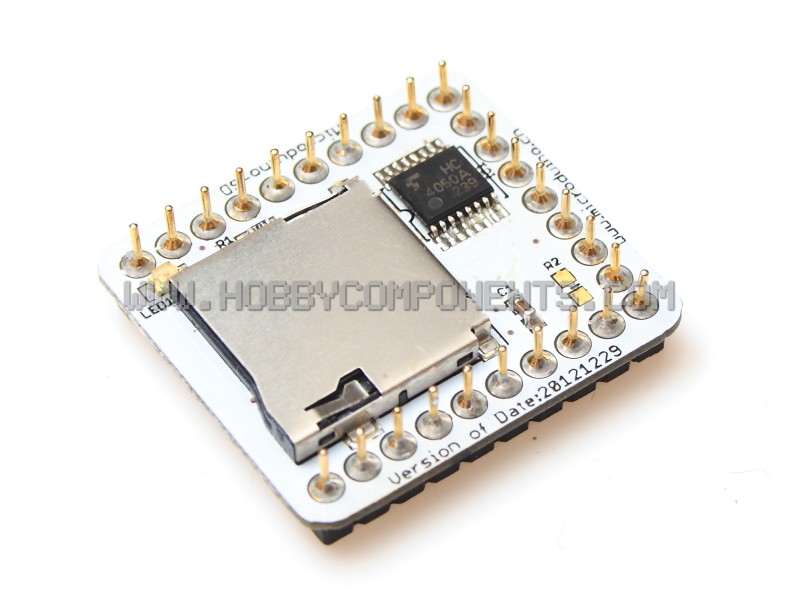
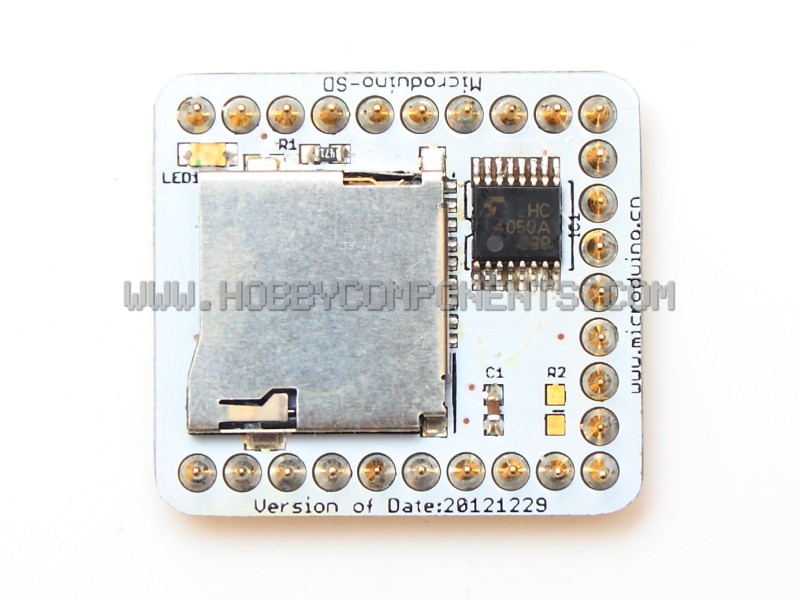
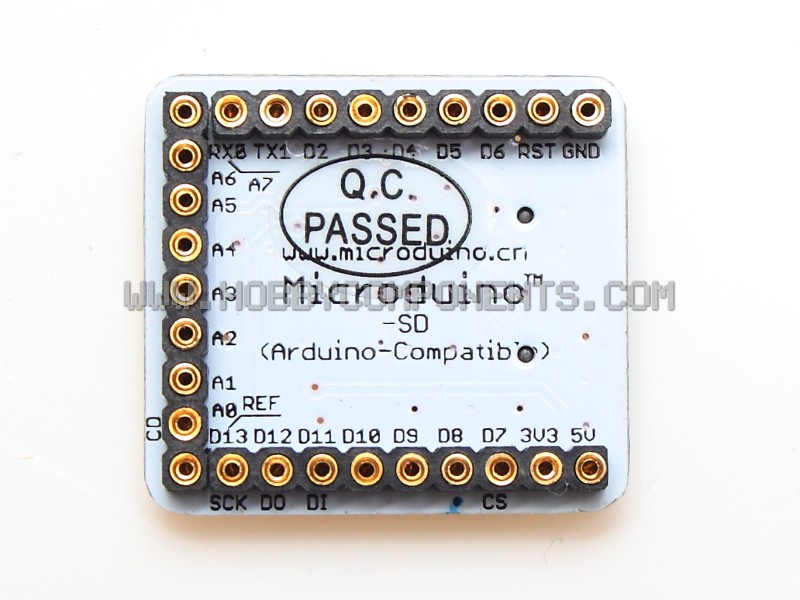
Description:
The Microduino-SD card module allows interface if micro SD and micro SDHC memory cards to the Microduino Core and Core+ boards.
Included on this module is a 5V to 3.3V level shifter to allow safe connection of the Core and Core+ 5V DIO pins to the micro Sd card. When used with the Microduino Core board this module works with the standard Arduino SD card library. If you with to use this module with the a Microduino Core+ board you will need to replace your SD card library with the one listed below.
SD Module Pin Connections:
CS..........D7
DO..........D11
DI...........D12
SCK.........D13
CD..........A0
IN...........3.3V
Microduino-SD Schematic:
Microduino-SD PCB:
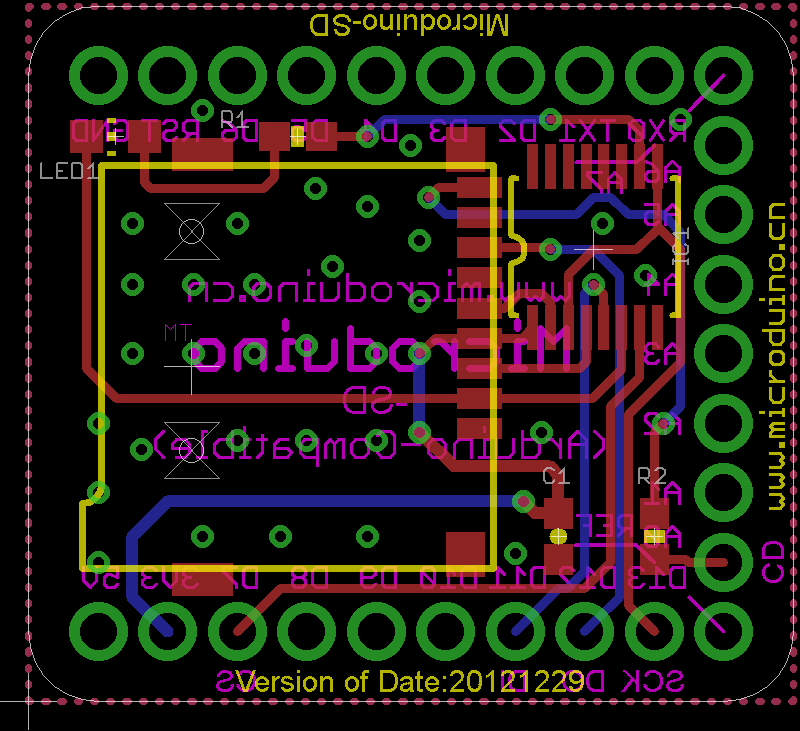
Microduino Core+ Library (not required for Core boards)
For Microduino Core+ boards you will need to download this updated SD library, unzip and rename its folder to SD, and use it to replace the one found in your \arduino\libraries\ folder
Example Write Sketch:
Code: Select all
/* FILE: Microduino_SD_CARD_MODULE_HCMIDU0009_Write_Example
DATE: 02/06/13
VERSION: 0.1
REVISIONS:
02/06/13 Created version 0.1
This is an example of how to use the Microduin SD card module (HCMIDU0009).
It allows reading and writing of data to a standard
micro SD card and is useful for applications such as data loggers where a large
amount of data needs to be stored. The module works with the standard
Arduino SD card library.
This example program will create a test file on the SD card called test.txt
If the file already exists it will first delete it and then create a new
one.
You may copy, alter and reuse this code in any way you like, but please leave
reference to HobbyComponents.com in your comments if you redistribute this code.
This software may not be used directly for the purpose of selling products that
directly compete with Hobby Components Ltd's own range of products.
THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO WARRANTIES, WHETHER
EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF
MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR LACK OF NEGLIGENCE.
HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR ANY DAMAGES,
INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR ANY
REASON WHATSOEVER.
*/
/* Include the standard SD card library */
#include <SD.h>
#define SD_CARD_CD_DIO 7 /* DIO pin used to control the modules CS pin */
File SDFileData;
/* Initialise serial*/
void setup()
{
Serial.begin(9600);
}
/* Main program loop */
void loop()
{
/* Initialise the SD card */
if (!SD.begin(SD_CARD_CD_DIO))
{
/* If there was an error output this to the serial port and go no further */
Serial.println("ERROR: SD card failed to initiliase");
while(1);
}else
{
Serial.println("SD Card OK");
}
/* Check if the text file already exists */
while(SD.exists("test.txt"))
{
/* If so then delete it */
Serial.println("test.txt already exists...DELETING");
SD.remove("test.txt");
}
/* Create a new text file on the SD card */
Serial.println("Creating test.txt");
SDFileData = SD.open("test.txt", FILE_WRITE);
/* If the file was created ok then add come content */
if (SDFileData)
{
SDFileData.println("It worked !!!");
/* Close the file */
SDFileData.close();
Serial.println("done.");
}else
{
Serial.println("Error writing to file !");
}
/* Do nothing */
while (1);
}
Example Read Sketch:
Code: Select all
/* FILE: Microduino_SD_CARD_MODULE_HCMIDU0009_Read_Example
DATE: 02/06/13
VERSION: 0.1
REVISIONS:
02/06/13 Created version 0.1
This is an example of how to use the Microduin SD card module (HCMIDU0009).
It allows reading and writing of data to a standard
micro SD card and is useful for applications such as data loggers where a large
amount of data needs to be stored. The module works with the standard
Arduino SD card library.
This example program will attempt to read a text file named text.txt and
output its contents to the serial port.
You may copy, alter and reuse this code in any way you like, but please leave
reference to HobbyComponents.com in your comments if you redistribute this code.
This software may not be used directly for the purpose of selling products that
directly compete with Hobby Components Ltd's own range of products.
THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO WARRANTIES, WHETHER
EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF
MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR LACK OF NEGLIGENCE.
HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR ANY DAMAGES,
INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR ANY
REASON WHATSOEVER. */
/* Include the standard SD card library */
#include <SD.h>
#define SD_CARD_CD_DIO 7 /* DIO pin used to control the modules CS pin */
File SDFileData;
/* Initialise serial */
void setup()
{
Serial.begin(9600);
}
/* Main program loop */
void loop()
{
/* Initiliase the SD card */
if (!SD.begin(SD_CARD_CD_DIO))
{
/* If there was an error output this to the serial port and go no further */
Serial.println("ERROR: SD card failed to initiliase");
while(1);
}else
{
Serial.println("SD Card OK");
}
/* Check if the text file exists */
if(SD.exists("test.txt"))
{
Serial.println("test.txt exists, attempting to read file...");
/* The file exists so open it */
SDFileData = SD.open("test.txt");
/* Sequentially read the data from the file and output it's
contents to the UART */
while (SDFileData.available())
{
Serial.write(SDFileData.read());
}
/* Close the file */
SDFileData.close();
}
/* Do nothing */
while (1);
}