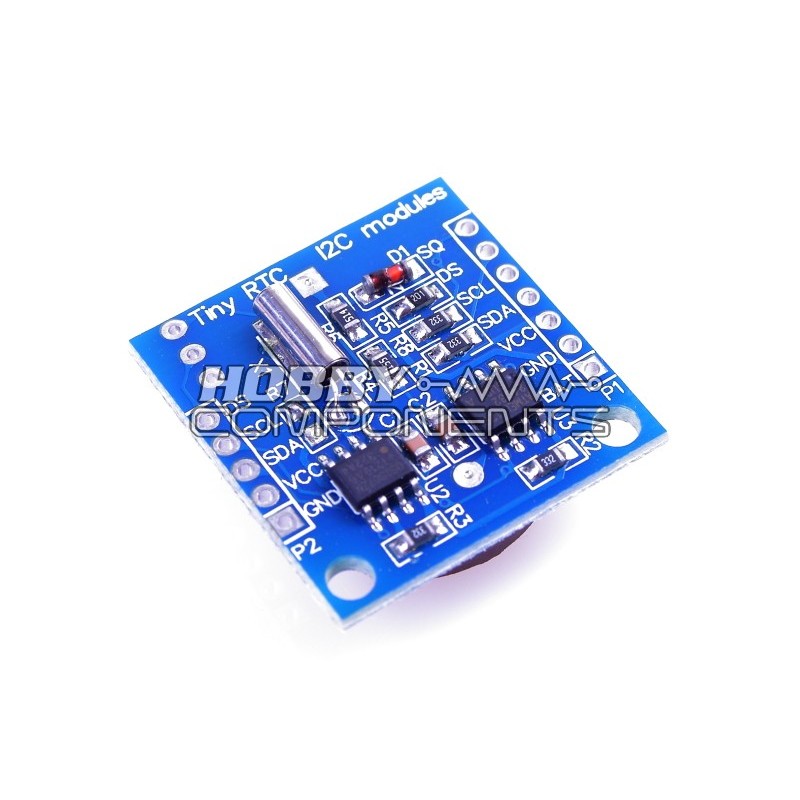
Real Time Clock module HCMODU0011
Description:
This Arduino library has been written to allow easy use DS1307 based real time clock modules such as our RTC module HCMODU0011. The library also contains functions to read and write to EEPROM memory which is also included in this RTC module. Communication with the module is via the standard Arduino I2C interface on pins A4 (SDA) & A5 (SCL) for an Uno, or 20 (SDA) & 21 (SCL) for a Mega.
You will need to download (please log in to download the library) and unzip this library to the Aduino development environments library area
On Windows:
My Documents\Arduino\libraries\
My Documents\Arduino\libraries\
My Documents\Arduino\libraries\
On Mac:
Documents/Arduino/libraries/
Documents/Arduino/libraries/
Documents/Arduino/libraries/
or similarly for Linux.
To use the library just include the Wire.h and HCRTC.h libraries and create an instance of the HCRTC library. E.g:
You can set the date and time with the following library function:HCRTC HCRTC;
And read the current date and time with the following library function:HCRTC.RTCWrite(I2C address, Year, Month, Day, Hour, Minuite, Second, Day of week);
Once the data has been read from the RTC module it can be accessed with the following library functions which return one byte of data of type byte:HCRTC.RTCRead(I2C address);
Or using the following string functions which return the time and date as a formatted string:HCRTC.GetDay()
HCRTC.GetMonth()
HCRTC.GetYear()
HCRTC.GetHour()
HCRTC.GetMinute()
HCRTC.GetSecond()
HCRTC.GetWeekday()
To write data (of type byte) to the modules EEPROM memory you can use the following sequence of library functions:HCRTC.GetTimestring()
HCRTC.GetDatestring()
Please note that when writing to the EEPROM, the data is temporarily stored in the EEPROM's cache memory until the HCRTC.EEEndWrite() function is executed. The maximum amount of data that this cache can hold in one write sequence is 64 bytes. You should therefore not attempt to write more than this ammount in one write sequence.HCRTC.EEStartWrite(EEPROM I2C address, EEPROM memory start location);
HCRTC.EEWriteByte(Data byte 0);
HCRTC.EEWriteByte(Data byte 1);
.
.
HCRTC.EEWriteByte(Data byte n);
HCRTC.EEEndWrite();
To read data (of type byte) from the modules EEPROM memory you can use the following read sequence:
HCRTC.EEStartRead(EEPROM I2C address, EEPROM memory start location);
Data_1 = HCRTC.EEReadByte(Data byte 0);
Data_2 = HCRTC.EEReadByte(Data byte 1);
.
.
Data_n = HCRTC.EEReadByte(Data byte n);
Example Code:
- /* FILE HCRTC_Example.cpp
- DATE: 23/12/13
- VERSION: 0.2
- AUTHOR: Andrew Davies
- This is an example of how to use the Hobby Components RTC library to read and
- write to and from the DS1307 real time clock 24C32 EEPROM. The library is intended
- to be used with our RTC clock module (HCMODU0011), but should work fine with any
- module that uses a DS1307 device.
- You may copy, alter and reuse this code in any way you like, but please leave
- reference to HobbyComponents.com in your comments if you redistribute this code.
- This software may not be used directly for the purpose of selling products that
- directly compete with Hobby Components Ltd's own range of products.
- THIS SOFTWARE IS PROVIDED "AS IS". HOBBY COMPONENTS MAKES NO WARRANTIES, WHETHER
- EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF
- MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE, ACCURACY OR LACK OF NEGLIGENCE.
- HOBBY COMPONENTS SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR ANY DAMAGES,
- INCLUDING, BUT NOT LIMITED TO, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR ANY
- REASON WHATSOEVER.
- */
- /* Include the wire library */
- #include "Wire.h"
- /* Include the Hobby Components RTC library */
- #include <HCRTC.h>
- /* Define the I2C addresses for the RTC and EEPROM */
- #define I2CDS1307Add 0x68
- #define I2C24C32Add 0x50
- /* Example string to write to EEPROM */
- char ExampleString[] = "Hobby Components";
- /* Used in example as an index pointer */
- byte index;
- /* Create an instance of HCRTC library */
- HCRTC HCRTC;
- void setup()
- {
- Serial.begin(9600);
- /* Use the RTCWrite library function to set the time and date.
- Parameters are: I2C address, year, month, date, hour, minute, second,
- day of week */
- HCRTC.RTCWrite(I2CDS1307Add, 13, 4, 24, 14, 21, 0, 3);
- }
- void loop()
- {
- /************* Example write to EEPROM ***************/
- /* Initiate a write sequence.
- Parameters are: I2C address, EEPROM start location */
- HCRTC.EEStartWrite(I2C24C32Add, 32000);
- for (index = 0; index < sizeof(ExampleString); index++)
- {
- /* Sequentially write example data to the EEPROM */
- HCRTC.EEWriteByte(ExampleString[index]);
- }
- /* End the write sequence */
- HCRTC.EEEndWrite();
- /* Wait for cached data to finish writing */
- delay(10);
- /************* Example read from EEPROM ***************/
- /* Initiate read sequence.
- Parameters are: I2C address, EEPROM start location */
- HCRTC.EEStartRead(I2C24C32Add, 32000);
- for (index = 0; index < sizeof(ExampleString); index++)
- {
- /* Sequentially read data from EEPROM and output it to the UART */
- Serial.write(HCRTC.EEReadByte(I2C24C32Add));
- }
- Serial.println();
- /* Continuously read the current time and date from the RTC */
- while(true)
- {
- /* Read the current time from the RTC module */
- HCRTC.RTCRead(I2CDS1307Add);
- /* Output the information to the UART */
- Serial.print(HCRTC.GetDay());
- Serial.print("/");
- Serial.print(HCRTC.GetMonth());
- Serial.print("/");
- Serial.print(HCRTC.GetYear());
- Serial.print(" ");
- Serial.print(HCRTC.GetHour());
- Serial.print(":");
- Serial.print(HCRTC.GetMinute());
- Serial.print(":");
- Serial.print(HCRTC.GetSecond());
- Serial.print(" DOW:");
- Serial.println(HCRTC.GetWeekday());
- /* Now output the same thing but using string functions instead: */
- Serial.print(HCRTC.GetDatestring());
- Serial.print(" ");
- Serial.println(HCRTC.GetTimestring());
- /* Wait a second before reading again */
- delay(1000);
- }
- }
Library:
Latest version (0.4)
Older versions:
V0.3
Diagrams, libraries, and example code are provided as an additional free service by Hobby Components and are not sold as part of this product. We do no provide any guarantees or warranties as to their accuracy or fitness for purpose.
Descriptions and diagrams on this page are copyright Hobby Components Ltd and may not be reproduced without permission.